
How to use JavaScript Arrays: A Comprehensive Guide
This is your ultimate guide to JavaScript arrays. You will learn how to create, add, remove, filter, map, and reduce arrays and you become a javascript arrays master ๐งโโ๏ธโจ.
What is an Array?
An array is a data structure that stores a collection of elements. Each element in an array has a unique index that allows you to access it.
For example, imagine you need to store a collection of fruits in a box, this box has 4 compartments and you can store a fruit in each compartment and each compartment has a unique number that allows you to access the fruit in it.
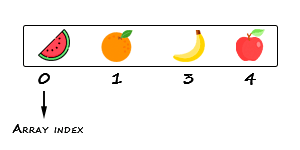
And this is how you can represent it in code:
1// ๐ is at index 0 2const oranges = ["๐", "๐", "๐", "๐"]; 3
How to create an Array in JavaScript ?
You can create an array in different ways in JavaScript. The most common way is by using the array literal syntax, but there are other ways to create an array.
1// Array literal syntax 2const fruits = ["๐", "๐", "๐", "๐"]; 3 4// Another way to create an array is by using the `Array` constructor: 5const fruits = new Array("๐", "๐", "๐", "๐") 6
There are some other advanced ways to create arrays, for example, you can create an array with a fixed length and later fill it with values:
1// Create an array with a fixed length of 4 2const fruits = new Array(4); 3 4// Fill the array with values 5fruits.fill("๐"); // ['๐', '๐', '๐', '๐'] 6 7// creating an array from other collections like a string 8const fruits = Array.from("๐๐๐๐"); // ['๐', '๐', '๐', '๐'] 9
How to access elements in an Array?
Let me show you how to access elements in an array with an example, imagine you have an array of fruits:
You can acces using the index of the element, in almost all programming languages the index starts at 0, so the first element in the array has an index of 0:
1const fruits = ["๐", "๐", "๐", "๐"]; 2 3// this is the static way to access elements in an array 4console.log(fruits[0]); // ๐ 5console.log(fruits[1]); // ๐ 6console.log(fruits[2]); // ๐ 7console.log(fruits[3]); // ๐ 8
In recent years in ES2022 you can use at()
method to access elements in an array:
1console.log(fruits.at(0)); // ๐ 2console.log(fruits.at(1)); // ๐ 3console.log(fruits.at(2)); // ๐ 4console.log(fruits.at(3)); // ๐ 5
As you can see, you can access elements in an array using the index of the element similar to static way but at()
is slightly different, let see:
1const fruits = ["๐", "๐", "๐", "๐"]; 2 3console.log(fruits.at(-1)); // ๐ 4console.log(fruits[-1]); // undefined 5
The at()
method allows you to access elements in an array using negative indexes, which is not possible with the static way, it is doing in circular way.
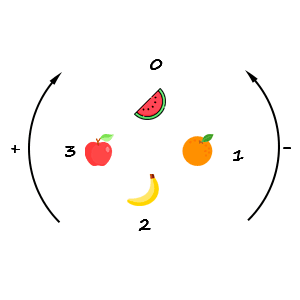
In the example we are accessing the last elment because it is one position to the left (negative) of the first element, in this case the element in -1
is ๐
.
Using the static way to access elements in an array with a negative index will return undefined
because it does not exist that position.
How to get an element without an index?
You can get an element from an array without knowing its index using the find()
method.
1const fruits = ["๐", "๐", "๐", "๐"]; 2const fruit = fruits.find(fruit => fruit === "๐"); 3// The fruit "๐" 4
In the above example, the condition to find the element is fruit === "๐"
, the find()
method returns the first element that satisfies the condition.
We can also use more sofisticated conditions to find elements in an array, let's see how to do it.
1const ages = [20, 30, 40, 50]; 2const age = ages.find(age => age > 30); 3// The age 40 4
But we also can get the index of an element without knowing it using the findIndex()
method similar to find()
method but in this case it returns the index of the element.
1const fruits = ["๐", "๐", "๐", "๐"]; 2const index = fruits.findIndex(fruit => fruit === "๐"); 3// The index 1 4
How to add elements to an Array?
You can add elements to an array using different methods, the most common methods are push()
, unshift()
, and splice()
.
Using push()
method
The push()
method adds one or more elements to the end of an array and returns the new length of the array.
1const fruits = ["๐", "๐", "๐", "๐"]; 2fruits.push("๐ฅฆ"); // length 5 3// The mutated array ["๐", "๐", "๐", "๐", "๐ฅฆ"] 4
Using unshift()
method
The unshift()
method adds one or more elements to the beginning of an array and returns the new length of the array.
1const fruits = ["๐", "๐", "๐", "๐"]; 2fruits.unshift("๐ฅฆ"); // length 5 3// The mutated array ["๐ฅฆ", "๐", "๐", "๐", "๐"] 4
Using splice()
method
The splice()
method adds or removes elements from an array. You can use it to add elements to an array at a specific index.
1const fruits = ["๐", "๐", "๐", "๐"]; 2fruits.splice(2, 0, "๐ฅฆ"); // [] 3// The mutated array ["๐", "๐", "๐ฅฆ", "๐", "๐"] 4
In the example, the splice()
method adds the element ๐ฅฆ
at index 2, the second argument 0
is the number of elements to remove, in this case, we are not removing any element.
So now, you know how to add elements to an array, at the beginning, at the end, or at a specific index, let's learn how to remove elements from an array.
How to remove elements from an Array?
You can remove elements from an array using different methods, the most common methods are pop()
, shift()
, and splice()
.
Using pop()
method
The pop()
method removes the last element from an array and returns the removed element.
1const fruits = ["๐", "๐", "๐", "๐"]; 2fruits.pop(); // "๐" 3// The mutated array ["๐", "๐", "๐"] 4
Using shift()
method
The shift()
method removes the first element from an array and returns the removed element.
1const fruits = ["๐", "๐", "๐", "๐"]; 2fruits.shift(); // "๐" 3// The mutated array ["๐", "๐", "๐"] 4
Using splice()
method
The splice()
method can also be used to remove elements from an array. You can use it to remove elements from a specific index.
1const fruits = ["๐", "๐", "๐", "๐"]; 2fruits.splice(2, 1); // ["๐"] 3// The mutated array ["๐", "๐", "๐"] 4
In the example, the splice()
method removes the element at index 2, the second argument 1
is the number of elements to remove, in this case, we are removing one element.
We can also remove elements using the filter()
method, let's see how to do it.
How to filter elements in an Array?
The filter()
method creates a new array with all elements that pass the test implemented by the provided function.
1const fruits = ["๐", "๐", "๐", "๐"]; 2const filteredFruits = fruits.filter(fruit => fruit !== "๐"); 3// The filtered array ["๐", "๐", "๐"] 4
This is one use case of the filter()
method, but in general you can use it to filter elements based on any condition and return a new transformed array from the original.
How to transforme an Array?
There are several methods in JavaScript that allow you to transform an array, we can get a new array filtered with the filter()
method, we can get a new array with the elements transformed with the map()
method, and we can get a single value from an array with the reduce()
method.
How to use the map()
method?
The map()
method creates a new array with the results of calling a provided function on every element in the array.
Lets map the fruits into fresh fruits with map methods:
1const fruits = ["๐", "๐", "๐", "๐"]; 2const transformedFruits = fruits.map(fruit => `โจ${fruit}โจ`); 3// The transformed array ["โจ๐โจ", "โจ๐โจ", "โจ๐โจ", "โจ๐โจ"] 4
Obviously in the real world you can do more complex transformations with the map()
method, you can transform the elements in any way you want.
How to use the reduce()
method?
The reduce()
method executes a reducer function on each element of the array, resulting in a single output value.
Let's reduce the fruits into a single string with the reduce method:
1const fruits = ["๐", "๐", "๐", "๐"]; 2const reducedFruits = fruits.reduce((acc, fruit) => `${acc} ${fruit}`, ""); 3// The reduced value " ๐ ๐ ๐ ๐" 4
In the example, the reduce()
method concatenates all the fruits into a single string, the initial value of the accumulator is an empty string ""
and the reducer function concatenates the accumulator with each fruit, the result is a new accumulated string that is returned on each iteration.
Let's see how to use the reduce()
method to sum all the elements in an array:
1const numbers = [1, 2, 3, 4, 5]; 2const sum = numbers.reduce((acc, number) => acc + number, 0); 3// 1 + 2 +3 + 4 + 5 = The sum 15 4
There are other methods in javascript arrays, but these are the most common methods that you will use in your day-to-day work.
But let's see some other methods that you can use to manipulate arrays and are useful in some cases.
How to sort elements in an Array?
You can sort elements in an array using the sort()
method.
1const numbes = ["0", "-1", "10", "2"]; 2const sortedNumbers = numbes.sort((a, b) => a - b); 3// The sorted array ["-1", "0", "2", "10"] 4
In the example, the sort()
method sorts the numbers in ascending order, the comparator function (a, b) => a - b
compares two numbers and returns a negative number if a
is less than b
, a positive number if a
is greater than b
, and zero if a
is equal to b
.
You can also sort elements in descending order by changing the comparator function to (a, b) => b - a
.
Finally there are 2 other methods I like to use in arrays, the every()
and some()
methods, that personally I use them a lot in my day-to-day work.
How to check if all elements in an Array pass a test?
The every()
method tests whether all elements in the array pass the test implemented by the provided function.
1const numbers = [1, 2, 3, 4, 5]; 2const allNumbersArePositive = numbers.every(number => number > 0); 3// The result is true 4
In the example, the every()
method checks if all numbers in the array are positive, the condition is number > 0
, and the result is true
because all numbers are positive.
How to check if some elements in an Array pass a test?
The some()
method tests whether at least one element in the array passes the test implemented by the provided function.
1const numbers = [1, 2, 3, 4, 5]; 2const someNumbersAreEven = numbers.some(number => number % 2 === 0); 3// The result is true 4
In the example, the some()
method checks if at least one number in the array is even, the condition is number % 2 === 0
, and the result is true
because there are even numbers in the array.
Conclusion
You have learned how to create, add, remove, filter, map, and reduce arrays in JavaScript. You are now a JavaScript arrays master ๐งโโ๏ธโจ.
As everythig in programming, practice is the key to master arrays, so I encourage you to practice what you have learned in this guide and try to solve different problems using arrays.
Also take a look at the MDN Web Docs to learn more about arrays and the methods available in JavaScript.
I hope you enjoyed this guide and learned something new, if you have any questions or feedback, feel free to reach out to me on Andrew_GMx.